mirror of
https://invent.kde.org/network/falkon.git
synced 2024-09-23 10:42:11 +02:00
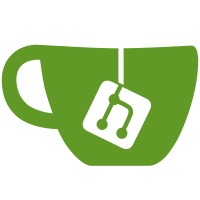
Summary: this is, for now, backwards compatible. in the long run a full move to QSP instead of manually doing path lookup would be good though. as that ideally means aligning capitalization between appname and on-disk paths we'll avoid that for now. fixes data lookup from XDG paths making the binary partially relocatable and properly configurable via XDG paths (e.g. corporate branding or what have you) Test Plan: - theme path resolution now walks all XDG paths Reviewers: drosca Reviewed By: drosca Differential Revision: https://phabricator.kde.org/D7600
166 lines
5.2 KiB
C++
166 lines
5.2 KiB
C++
/* ============================================================
|
|
* Falkon - Qt web browser
|
|
* Copyright (C) 2014-2017 David Rosca <nowrep@gmail.com>
|
|
*
|
|
* This program is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
* ============================================================ */
|
|
#include "datapaths.h"
|
|
#include "qztools.h"
|
|
|
|
#include <QApplication>
|
|
#include <QDir>
|
|
|
|
#include <QStandardPaths>
|
|
|
|
Q_GLOBAL_STATIC(DataPaths, qz_data_paths)
|
|
|
|
DataPaths::DataPaths()
|
|
{
|
|
init();
|
|
}
|
|
|
|
// static
|
|
void DataPaths::setCurrentProfilePath(const QString &profilePath)
|
|
{
|
|
qz_data_paths()->initCurrentProfile(profilePath);
|
|
}
|
|
|
|
// static
|
|
void DataPaths::setPortableVersion()
|
|
{
|
|
DataPaths* d = qz_data_paths();
|
|
d->m_paths[Config] = d->m_paths[AppData];
|
|
|
|
d->m_paths[Profiles] = d->m_paths[Config];
|
|
d->m_paths[Profiles].first().append(QLatin1String("/profiles"));
|
|
|
|
d->m_paths[Temp] = d->m_paths[Config];
|
|
d->m_paths[Temp].first().append(QLatin1String("/tmp"));
|
|
|
|
// Make sure the Config and Temp paths exists
|
|
QDir dir;
|
|
dir.mkpath(d->m_paths[Config].at(0));
|
|
dir.mkpath(d->m_paths[Temp].at(0));
|
|
}
|
|
|
|
// static
|
|
QString DataPaths::path(DataPaths::Path path)
|
|
{
|
|
Q_ASSERT(!qz_data_paths()->m_paths[path].isEmpty());
|
|
|
|
return qz_data_paths()->m_paths[path].at(0);
|
|
}
|
|
|
|
// static
|
|
QStringList DataPaths::allPaths(DataPaths::Path type)
|
|
{
|
|
Q_ASSERT(!qz_data_paths()->m_paths[type].isEmpty());
|
|
|
|
return qz_data_paths()->m_paths[type];
|
|
}
|
|
|
|
// static
|
|
QString DataPaths::currentProfilePath()
|
|
{
|
|
return path(CurrentProfile);
|
|
}
|
|
|
|
// static
|
|
void DataPaths::clearTempData()
|
|
{
|
|
QzTools::removeDir(path(Temp));
|
|
}
|
|
|
|
void DataPaths::init()
|
|
{
|
|
// AppData
|
|
#if defined(Q_OS_MACOS)
|
|
m_paths[AppData].append(QApplication::applicationDirPath() + QLatin1String("/../Resources"));
|
|
#elif defined(Q_OS_UNIX) && !defined(NO_SYSTEM_DATAPATH)
|
|
m_paths[AppData].append(USE_DATADIR);
|
|
#else
|
|
m_paths[AppData].append(QApplication::applicationDirPath());
|
|
#endif
|
|
|
|
m_paths[Translations].append(m_paths[AppData].at(0) + QLatin1String("/locale"));
|
|
m_paths[Themes].append(m_paths[AppData].at(0) + QLatin1String("/themes"));
|
|
m_paths[Plugins].append(m_paths[AppData].at(0) + QLatin1String("/plugins"));
|
|
|
|
// Add standard data lookup paths (our appname has a capital F so we manually construct
|
|
// the final paths for now)
|
|
for (auto location : QStandardPaths::standardLocations(QStandardPaths::GenericDataLocation)) {
|
|
m_paths[Translations].append(location + QLatin1String("/falkon/locale"));
|
|
m_paths[Themes].append(location + QLatin1String("/falkon/themes"));
|
|
m_paths[Plugins].append(location + QLatin1String("/falkon/plugins"));
|
|
}
|
|
|
|
// Config
|
|
#if defined(Q_OS_WIN) || defined(Q_OS_OS2)
|
|
// Use %LOCALAPPDATA%/falkon as Config path on Windows
|
|
m_paths[Config].append(QStandardPaths::writableLocation(QStandardPaths::AppLocalDataLocation));
|
|
#elif defined(Q_OS_MACOS)
|
|
m_paths[Config].append(QDir::homePath() + QLatin1String("/Library/Application Support/Falkon"));
|
|
#else // Unix
|
|
m_paths[Config].append(QStandardPaths::writableLocation(QStandardPaths::GenericConfigLocation) + QL1S("/falkon"));
|
|
#endif
|
|
|
|
// Profiles
|
|
m_paths[Profiles].append(m_paths[Config].at(0) + QLatin1String("/profiles"));
|
|
|
|
// Temp
|
|
#ifdef Q_OS_UNIX
|
|
const QByteArray &user = qgetenv("USER");
|
|
const QString &tempPath = QString(QSL("%1/falkon-%2")).arg(QDir::tempPath(), user.constData());
|
|
m_paths[Temp].append(tempPath);
|
|
#else
|
|
m_paths[Temp].append(m_paths[Config].at(0) + QLatin1String("/tmp"));
|
|
#endif
|
|
|
|
// Cache
|
|
#ifdef Q_OS_UNIX
|
|
const QString &cachePath = QStandardPaths::writableLocation(QStandardPaths::GenericCacheLocation);
|
|
if (!cachePath.isEmpty())
|
|
m_paths[Cache].append(cachePath + QLatin1String("/falkon"));
|
|
#endif
|
|
|
|
// Make sure the Config and Temp paths exists
|
|
QDir dir;
|
|
dir.mkpath(m_paths[Config].at(0));
|
|
dir.mkpath(m_paths[Temp].at(0));
|
|
|
|
// We also allow to load data from Config path
|
|
m_paths[Translations].append(m_paths[Config].at(0) + QLatin1String("/locale"));
|
|
m_paths[Themes].append(m_paths[Config].at(0) + QLatin1String("/themes"));
|
|
m_paths[Plugins].append(m_paths[Config].at(0) + QLatin1String("/plugins"));
|
|
|
|
#ifdef USE_LIBPATH
|
|
m_paths[Plugins].append(QLatin1String(USE_LIBPATH "/falkon"));
|
|
#endif
|
|
}
|
|
|
|
void DataPaths::initCurrentProfile(const QString &profilePath)
|
|
{
|
|
m_paths[CurrentProfile].append(profilePath);
|
|
|
|
if (m_paths[Cache].isEmpty())
|
|
m_paths[Cache].append(m_paths[CurrentProfile].at(0) + QLatin1String("/cache"));
|
|
|
|
if (m_paths[Sessions].isEmpty())
|
|
m_paths[Sessions].append(m_paths[CurrentProfile].at(0) + QLatin1String("/sessions"));
|
|
|
|
QDir dir;
|
|
dir.mkpath(m_paths[Cache].at(0));
|
|
dir.mkpath(m_paths[Sessions].at(0));
|
|
}
|